11 More About Matrices
Now that you have seen some ways to create matrices, let’s discuss a number of basic manipulations of matrices. I will show you examples of various operations, and then you’ll have the chance to put them in practice with some exercises listed at the end of the chapter.
11.1 Basic Operations with Matrices
- Selecting elements:
- select a given cell
- select a set of cells
- select a given row
- select a set of rows
- select a given column
- select a set of columns
- Adding a new column
- Adding a new row
- Deleting a column
- Deleting a row
- Renaming a column
- Moving a column
Let’s say you have a matrix mat
with the following content:
# inputs
= 1000
deposit = 0.02
rate_savings = 0.025
rate_moneymkt = 0.03
rate_certificate = 0:5
years
# future values
= deposit * (1 + rate_savings)^years
savings = deposit * (1 + rate_moneymkt)^years
moneymkt = deposit * (1 + rate_certificate)^years
certificate
# matrix
= matrix(c(years, savings, moneymkt, certificate), nrow = 6, ncol = 4)
mat
# row and columns names
rownames(mat) = 1:6
colnames(mat) = c("year", "savings", "moneymkt", "certificate")
mat
year savings moneymkt certificate1 0 1000.000 1000.000 1000.000
2 1 1020.000 1025.000 1030.000
3 2 1040.400 1050.625 1060.900
4 3 1061.208 1076.891 1092.727
5 4 1082.432 1103.813 1125.509
6 5 1104.081 1131.408 1159.274
11.1.1 Selecting elements
The matrix mat
is a 2-dimensional object: the 1st dimension corresponds
to the rows, while the 2nd dimension corresponds to the columns.
Because mat
has two dimensions, the bracket notation involves
working with data frames in this form: mat[ , ]
.
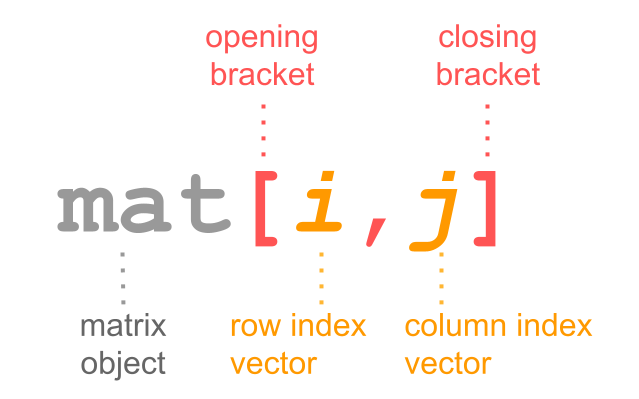
Figure 11.1: Bracket notation in matrices
In other words, you have to specify values inside the
brackets for the 1st index, and the 2nd index: mat[index1, index2]
.
Selecting cells
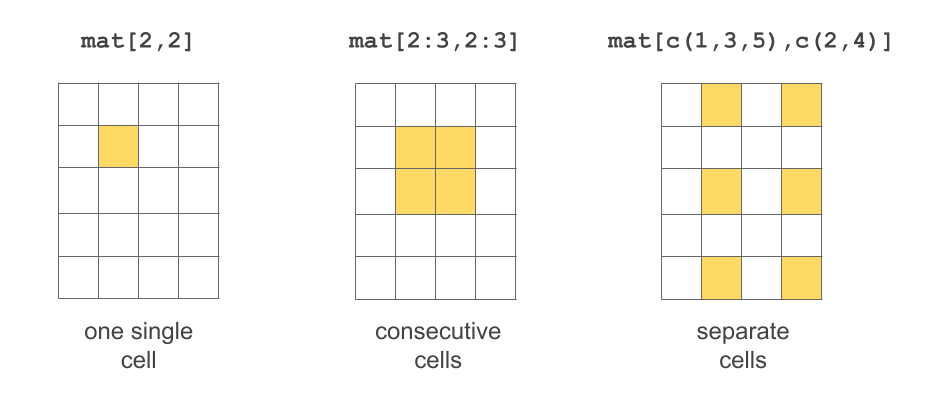
Figure 11.2: Several ways to select cells
# select value in row 1 and column 1
1, 1]
mat[> [1] 0
# select value in row 2 and column 3
2, 3]
mat[> [1] 1025
# select values in these cells
1:2, 3:4]
mat[> moneymkt certificate
> 1 1000 1000
> 2 1025 1030
It is also possible to exclude certain rows-and-columns by passing negative numeric indices:
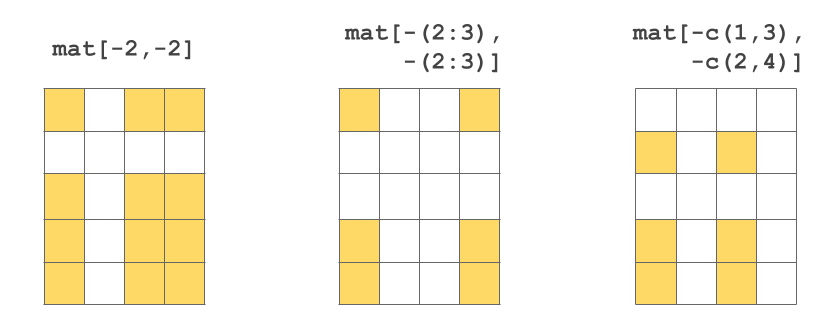
Figure 11.3: Several ways to exclude cells
Selecting rows
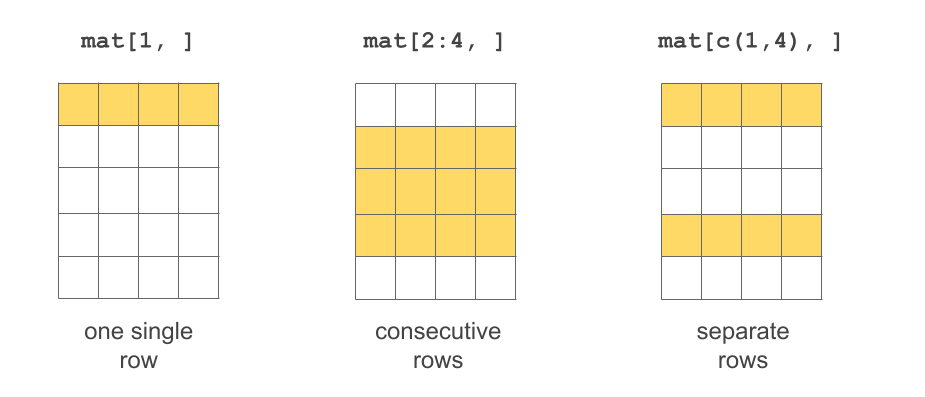
Figure 11.4: Several ways to select rows
If no value is specified for index1
then all rows are included. Likewise,
if no value is specified for index2
then all columns are included.
# selecting first row
1, ]
mat[> year savings moneymkt certificate
> 0 1000 1000 1000
# selecting third row
3, ]
mat[> year savings moneymkt certificate
> 2.000 1040.400 1050.625 1060.900
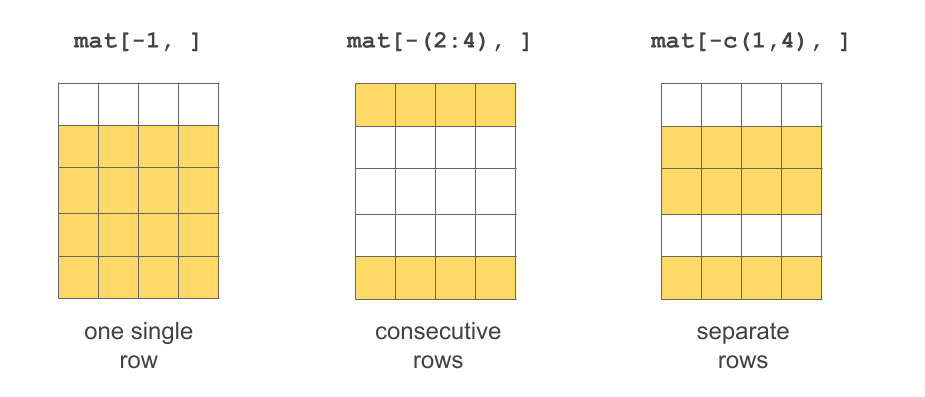
Figure 11.5: Several ways to exclude rows
Selecting columns
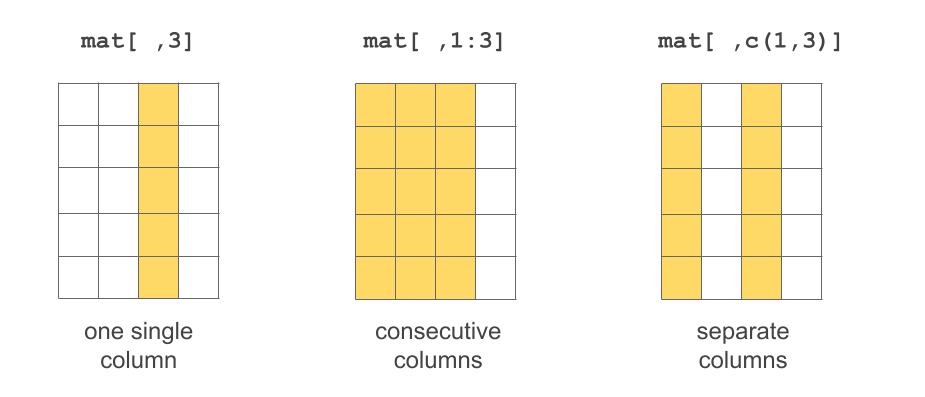
Figure 11.6: Several ways to select columns
# selecting second column
2]
mat[ ,> 1 2 3 4 5
> 1000.000 1020.000 1040.400 1061.208 1082.432
> 6
> 1104.081
# selecting columns 2 to 4
2:4]
mat[ ,> savings moneymkt certificate
> 1 1000.000 1000.000 1000.000
> 2 1020.000 1025.000 1030.000
> 3 1040.400 1050.625 1060.900
> 4 1061.208 1076.891 1092.727
> 5 1082.432 1103.813 1125.509
> 6 1104.081 1131.408 1159.274
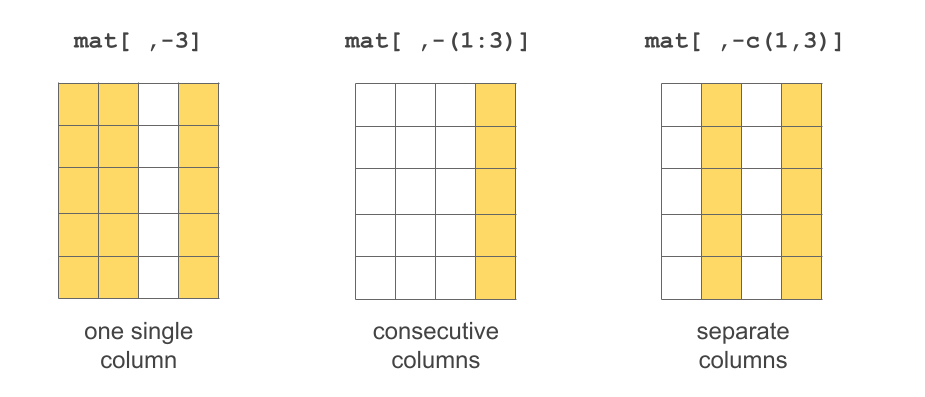
Figure 11.7: Several ways to exclude columns
11.1.2 Adding a column
To add a column, use the column-bind function cbind()
# vector
<- c(2, 4, 6, 8, 10, 12)
vec
# adding weights to mat
<- cbind(mat, vec)
mat
mat> year savings moneymkt certificate vec
> 1 0 1000.000 1000.000 1000.000 2
> 2 1 1020.000 1025.000 1030.000 4
> 3 2 1040.400 1050.625 1060.900 6
> 4 3 1061.208 1076.891 1092.727 8
> 5 4 1082.432 1103.813 1125.509 10
> 6 5 1104.081 1131.408 1159.274 12
11.1.3 Deleting a column
What if you want to delete a column? Simple: use a negative index to exclude the undesired column(s)
# deleting fifth column
<- mat[ ,-5]
mat
mat> year savings moneymkt certificate
> 1 0 1000.000 1000.000 1000.000
> 2 1 1020.000 1025.000 1030.000
> 3 2 1040.400 1050.625 1060.900
> 4 3 1061.208 1076.891 1092.727
> 5 4 1082.432 1103.813 1125.509
> 6 5 1104.081 1131.408 1159.274
11.1.4 Moving a column
What if you want to move one or more columns to a different position? For
example, what if you want to move year
to the last position (last column)?
One option is to create a vector of column indices in the desired order, and then
use this vector (for the index of columns) to reassign the matrix like this:
<- c(2:4, 1)
reordered_cols <- mat[ ,reordered_cols]
mat
mat> savings moneymkt certificate year
> 1 1000.000 1000.000 1000.000 0
> 2 1020.000 1025.000 1030.000 1
> 3 1040.400 1050.625 1060.900 2
> 4 1061.208 1076.891 1092.727 3
> 5 1082.432 1103.813 1125.509 4
> 6 1104.081 1131.408 1159.274 5